It’s far too late for any list of WWDC wants and wishes to have any actual effect on what is released. This is my short list of what I’d love to see added to SwiftUI and Xcode.
If you want to see features and fixes you need to file a Radar. It's the only way to communicate directly with Apple's engineering team about the changes you'd like to see in their products.
It's a myth that Radar/Feedback has no effect or is a blackhole. I worked daily with Radars while I was a writer at Apple, and worked on Radar itself as a contractor. Radars drive bug fixes and Anika will thank you.
For SwiftUI
There is definitely room for more controls in SwiftUI and these are some of the Views and ViewModifiers that I’d love to see.
A Wrapping HStack
It’s common to have an array of items, and want to display them horizontally, but have them wrap to multiple lines. For example, a number of tokens or hashtags shown within capsules as below.
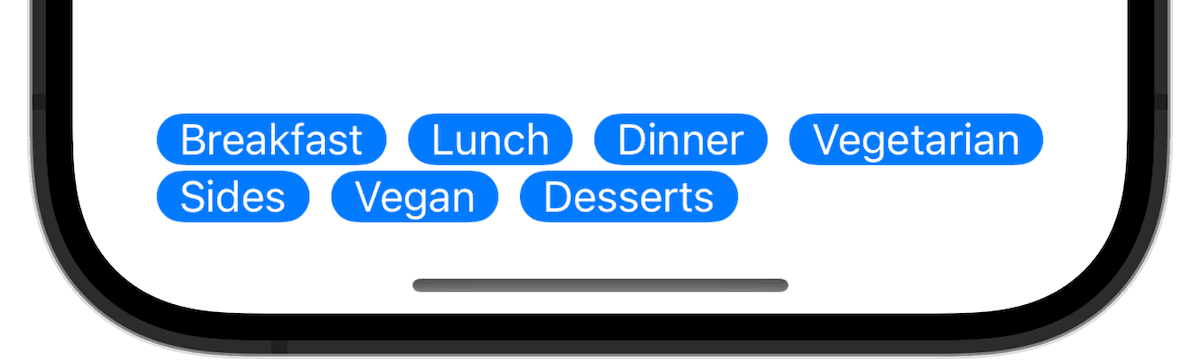
Button
viewsRight now you’re able to accomplish this through a couple of different means. You can do the math yourself, determining the width of each item, and then calculating how many will fit in row. From there figure out how many rows you’ll wide up with. Then simply create a VStack
with individual HStacks
for each of the pre-calculated sets of date. This works. although requires a bunch of effort.
There may be another way of accomplishing the same thing using Layout, but I’ve not explored that as depending on the latest iOS version is often out of reach for many apps.
NSTokenField
for SwiftUI
Along the same lines, it would be fantastic to get a native, or wrapped, version of the NSTokenField
implementation. You can get some of this functionality now, but only in a `.searchable` field.

Using .searchable
when dealing with a standard search field works well, but that's your only stock option.
NSTokenField on the other hand allows you to type in a word and make it into a token. This is similar to what Mail does, and the functionality that you might need when creating a query in a custom interface element.
General Class Wishes
Not SwiftUI specific, these additions would be useful for SwiftUI, iOS, and macOS development.
Type-safe strings for localization
The new string catalogs are great. But they're missing a couple of major issues. The first being no type-safe declarations. Yes, you can use Text(localized:"some.localized.string")
. But that will lead to those keys showing up in your app at some point.
This has been a few years coming, but Xcode now parses the colors and images in the assets catalog and provides type-safe references to them. Which is fantastic.
But we need something similar for localizable strings.
My personal solution was creating an open-source tool called Paracord. Paracord takes a YAML file of the form:
/**** No Parameters **/
/* General - plain_text */
"plain_text_all" = "Plain text - English";
/* General - plain text */
"plain_text_missing_spanish" = "Plain text - English";
And each additional language is in a separate file When you run the Paracord
tool, it generates an English Localized.strings
, as well as Localized.strings
provided files.
This in turn generates a Swift file of the format:
import Foundation
@objc
class Paracord: NSObject {
// MARK: No Parameters
/// Returns the localized string for the key `plain_text_all` - "Plain text - English"
/// Comment: "General - plain_text"
static var plainTextAll: String {
return localizedString(key: "plain_text_all")
}
}
When you need to refer to a string you use Text(Paracord.stringName)
.
If you strings have substitutions, a static func
is created in additional to the static var
, which takes the arguments. Note that the arguments are named. That's an option in the YAML so that you're able to reorder them for different languages and still know what they mean.
class Paracord {
/// Returns the user's birthday in the localized format.
/// - Parameters:
/// - name: The user's name.
/// - birthday: The user's birthday.
/// - time: The time of the user's birth.
/// - Returns: The localized string with the arguments in the specified format.
static func threeOrderedParametersWithName(name: String, birthday: String, time: String) -> String {
let formattedString = localizedString(key: "three_ordered_parameters_with_name")
let outputString = String(format: formattedString, name, birthday, time)
return outputString
}
}
And as there are full DocC comments in the code, the code completion is quite sweet.
An SFSymbol type-safe class
or enum
All these thousands of beautiful SFSymbols and not a type-safe way to refer to them. 😢
Without an Apple-provided solution, my personal approach to using SFSymbols is to create an enum
that maps cases to strings. The problem is that you need to add each of the SFSymbols you’re interested in being able to display. My stock implementation (kept in Xcode snippets) will return either a SwiftUI Image
and a UIImage
, but it would be much better if Apple gave us an up-to-date solution for this.
public enum SFSymbols: String, CaseIterable {
case clock = "clock"
case star = "star.fill"
/// Returns a UIkit `UIImage` instance.
public var uiImage: UIImage {
let image = UIImage(systemName: rawValue)
return image!
}
/// Returns a SwiftUI `Image` view.
public var image: Image {
return Image(systemName: rawValue)
}
The enum
is CaseIterable
so I can have a simple unit test that ensures that all the symbols are spelled correctly and available for the target OS version.
For Xcode
Xcode isn’t getting away without a few wishes of its own. I have a few of my own bugs that I’ve filed and would love to get fixed, but otherwise.
Better formatting control
The new Editor -> Structure -> Split Multiple Lines option under the Editor menu is at best awkward and at works almost impossible to read.
There is the member-wise init that Xcode generated for one of my classes:

init
for one of my classesThe Editor -> Structure -> Split Multiple Lines function currently reformats that code like this:
init(
id: String,
name: String,
category: String,
geographicArea: String,
instructions: String,
mealThumbnailURL: URL? = nil,
videoURL: URL? = nil,
ingredients: [Ingredient]?,
keywords: [String]?
) {
I much prefer this formatting.
init(id: String,
name: String,
category: String,
geographicArea: String,
instructions: String,
mealThumbnailURL: URL? = nil,
videoURL: URL? = nil,
ingredients: [Ingredient]?,
keywords: [String]?) {
I do understand that the current Xcode format allows for longer parameters, and can make a difference when it comes to resolving merge conflicts. Right now I need to install SwiftFormat and its plugin to do this any way other than manually. We have indentation options in Settings, please give us one for this.
Snippets sync to iCloud or Github
Xcode has a great snippets functionality. And I enjoy using it for a number of chunks of code I often use. But it would be so much better if it would synchronize to either iCloud or Github. Moving machines or using the same snippet within a team would much so much easier of this as an option.
Improvements to pbproj
to minimize merge conflicts
OK, this is an oldie, but a goodie. We need some sort of improvement to pbproj
so the dreaded "merge conflict in pbproj" is minimized. Perhaps that's what Apple's new PIKL will end up being used for.
I think just alphabetizing the list of files that aren't shown in the browser would make a big different.
Privacy Manifest code analysis
Donny Wals has created a very handy tool for determining the privacy settings that you'll need to set for submitting to Xcode. And it's fantastic.
But there is very little reason that Xcode couldn't analyze your code and generate a privacy manifest for you. AI and all.
Documentation
I spent 12 years of my life writing developer documentation at Apple and I loved every minute of it. I'd go back to doing it in a heartbeat.
But the current documentation is a mixed bag. Some of it is good, but a lot of it is missing. And it’s terribly hard to navigate.
Any reference material should have either pictures or sample code included. Especially when references often contain nothing more than init function with generics in them and links to other docs.
The new tutorials are far too concentrated on motion, which distracts users. It’s much better to provide it in a manner that can easily be copied and pasted.
And bring back Revision History, please.
Sample Code
All of the sample code that apple releases should be available on Apple’s GitHub. No exceptions. Right now it’s a hunt for anything you might need to find.